Python “Ping” Tool For Mac OS X
While I was studying some python to try to better understand how automation works and how python can speed up the jobs we do on our computers, I had the idea of making a quick python script for pinging IP addresses. I was lucky enough to find a quick, simple, easy to follow YouTube video that walked me through it, so it took out the thought of it all, only problem is… I’m the only person in history studying cyber security that uses a Mac. So with a little bit more research I learned how to change the code to make it work on Mac the same way that it was working on Windows systems. Mind you, since I altered the code to make it Mac specific, it will no longer work on Windows without altering it again.
If you are a Windows user and are interested in making this tool, it will take less than ten minutes, probably less than five. I will have the original video I got my code from here (if that link doesn’t work it will also be at the end of this article.
Anyway, here is the code for a simple ping tool that will run successfully on Mac:
#Ping.py — A Simple Script to check if IP address can receive packets
#Originally by WebDevPro — Adapted to work on Mac OS X
import os #Importing system specific commands
os.system(‘clear’) #CLS would be used for Win. Mac uses ‘clear’
print(“#” * 60) #Formatting
ip_target = input(“Target IP Address: “) #Get input of IP to ping
print(“-” * 60) #Formatting
os.system(‘ping -c 4 {}’.format(ip_target)) #Automating ping command that would normally be typed out in the console
print(“-” * 60) #Formatting
input(“Press any Key to Exit”) #Placeholder to end the program
Alright, super simple, right? Only 8 lines of code (excluding my comments). The only differences between this version and the version that would run on Windows is, the Windows version would be using ‘cls’ instead of ‘clear’ in line 4 and instead of ‘-c’ on line 8 would be ‘-n’.
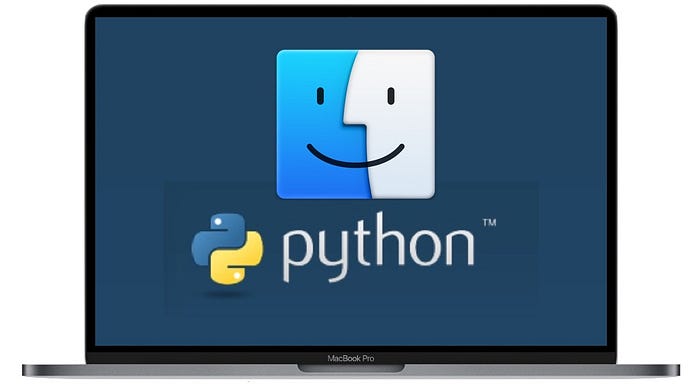
Explaining The Script
I believe my comments should make it easy enough to understand what is going on in this script but I’ll break it down a little bit more incase it isn’t clear enough.
Line 1 & 2 are explaining what this script does
Line 3 is telling python that we will be using the system commands that would normally be used in the Terminal (Mac) or Command Prompt (Windows)
Line 4 is simply clearing the screen so that when we are running this program we don’t have to see our terminal/command prompt screen flooded with whatever messages were previously on it
Line 5 draws a border for formatting purposes to keep everything looking clean and organized
Line 6 asks for input from the person running the script. This is where the user would type in the IP address that we would like to ping (or alternatively, the URL if we do not know the IP address).
Line 7 draws a border again for formatting
Line 8 is where the system performs the ping to the target IP address. The “4” in this line is telling the script to ping the target 4 times, you can change this however you would like but if I remember correctly, when I did not have a number in this line, it kept pinging as long as the script was running.
Line 9 draws a border again for formatting
Line 10 keeps the information on our screen until the user hits the “Enter” key. Yes, I know, my code says “Press Any Key”, and I am a liar.
I hope this helped any of you who are also, for some reason, using a Mac and also learning python. I would like to again restate what I said in the introduction of this article, I did not write the original code, and I am not taking credit for it. All I did was change two words to make the script function as designed on a Mac. All of the credit for the original code goes to WebDevPro on YouTube, and his original video can be found at the following: